Pair trading is a popular statistical arbitrage strategy that capitalizes on the price relationship between two co-integrated stocks. In this article, we will explore a powerful pair trading strategy using Dow Jones stocks. We will explain the code’s functions and the terminologies involved, with a focus on both the trading logic and the Python implementation.
For downloading many more Strategies Juypter Notebook, you can follow my Github repository Econometrics_for_Quants
Table of Contents
Understanding Pair Trading and Cointegration Analysis
Pair trading involves identifying two stocks that exhibit a strong historical correlation. However, instead of merely capitalizing on this correlation, the strategy aims to identify moments when the stock prices temporarily deviate from their usual relationship. These deviations present potential opportunities for profitable trades.
To identify eligible pairs for pair trading, we employ a critical statistical concept called cointegration analysis. This process determines whether two-time series move together in the long run despite temporary divergences. We perform cointegration analysis using the Augmented Dickey-Fuller (ADF) test, which calculates a p-value to assess the presence of a unit root in the spread between two stocks. If the p-value is less than 0.05, we consider the pair co-integrated, indicating that deviations between them tend to revert to their historical mean.
The Pair Trading Strategy Logic
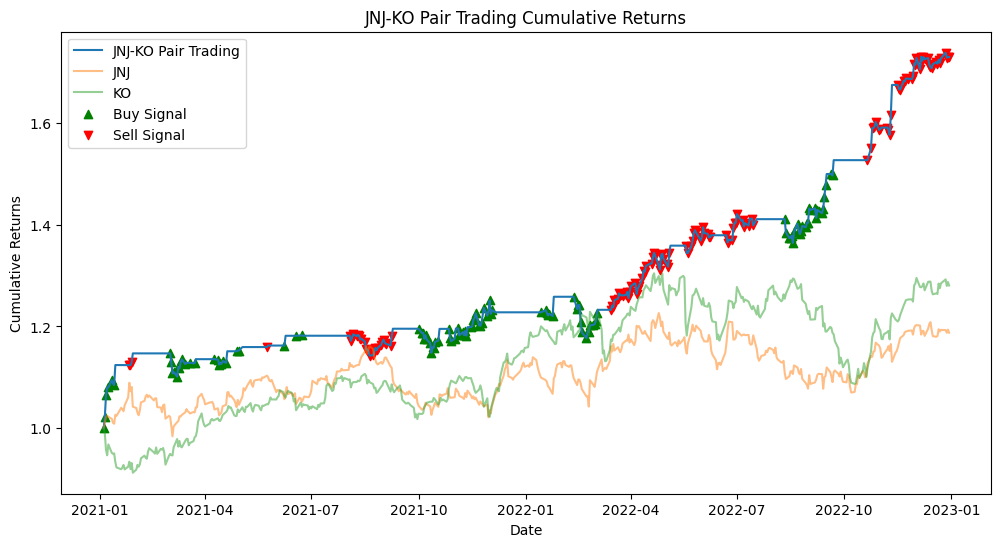
The pair trading strategy is designed to open a position when the spread between the two stocks diverges significantly from its historical mean. We use the z-score to measure this deviation:
Z-score = (Spread – Spread Mean) / Spread Standard Deviation
The z-score tells us how many standard deviations the spread is away from its historical mean. When the z-score exceeds a predefined entry threshold (e.g., 1.0), the strategy triggers a long or short signal, depending on the direction of the deviation.
However, we introduce the condition that the first position is only taken if the z-score reaches the entry threshold. Subsequent positions are taken only when the previous position is closed.
The strategy also includes an exit signal, which occurs when the z-score approaches zero or the returns in the next time interval turn negative. In these cases, the strategy closes the position, preventing potential losses when the spread converges or moves against the trader.
You can find Free Trading Strategies and much more content on my blog quantifiedtrader.com
The Python Code Implementation
To implement this strategy, we first fetch historical data for the Dow Jones stocks using the Yahoo Finance API. We then identify eligible pairs based on high correlation and conduct cointegration analysis using the ADF test.
import yfinance as yf
import statsmodels.tsa.stattools as ts
import pandas as pd
import matplotlib.pyplot as plt
from itertools import combinations
import warnings
warnings.filterwarnings("ignore")
# Define the Dow Jones stocks you want to analyze
dow_jones_tickers = [
"AAPL", "AMGN", "AXP", "BA", "CAT", "CRM", "CSCO", "CVX", "DIS", "DOW",
"GS", "HD", "HON", "IBM", "INTC", "JNJ", "JPM", "KO", "MCD", "MMM",
"MRK", "MSFT", "NKE", "PG", "TRV", "UNH", "V", "VZ", "WBA", "WMT",
"MMM", "HWM", "BA", "IBM", "TRV", "NKE", "DOW", "GS", "CAT", "CVX",
"MSFT", "DIS", "MCD", "V", "UNH", "JPM", "HD", "AAPL", "HON", "WMT",
"INTC", "PG", "AMGN", "WBA", "JNJ", "CSCO", "CRM", "KO"
]
# Fetch historical data for the Dow Jones stocks
data = yf.download(dow_jones_tickers, start="2021-01-01", end="2023-01-01")["Adj Close"]
# Find pairs of stocks with high correlation
pairs = []
correlation_threshold = 0.75
for stock1, stock2 in combinations(dow_jones_tickers, 2):
if stock1 != stock2: # Remove pairs with themselves
correlation = data[stock1].corr(data[stock2])
if correlation > correlation_threshold:
pair = tuple(sorted([stock1, stock2])) # Sort to eliminate duplicates
if pair not in pairs:
pairs.append(pair)
# Perform cointegration analysis
for pair in pairs:
stock1 = pair[0]
stock2 = pair[1]
cointegration_test = ts.coint(data[stock1], data[stock2])
p_value = cointegration_test[1]
if p_value < 0.05:
cointegration_results = cointegration_results.append(
{"Pair": f"{stock1}-{stock2}", "Cointegration P-Value": p_value},
ignore_index=True)
# Initialize an empty DataFrame to store cointegration test results
cointegration_results = pd.DataFrame(cointegration_results,columns=["Pair", "Cointegration P-Value"])
cointegration_results
# Print eligible pairs for pair trading
print("Eligible Pairs for Pair Trading:")
for idx, row in cointegration_results.iterrows():
print(row["Pair"])
# Plot cumulative returns, stocks' returns, and buy/sell signals for selected pairs
pairs_to_plot = cointegration_results["Pair"].head(5) # Plot first 5 pairs for illustration
Next, we create the pair_trading_strategy
function. This function calculates the spread between the two stocks, computes the z-score, and determines when to open and close positions based on the defined thresholds and conditions.
# Implement pair trading strategy and plot cumulative returns for selected pairs
def pair_trading_strategy(data, pair):
stock1 = pair[0]
stock2 = pair[1]
spread = data[stock1] - data[stock2]
spread_mean = spread.mean()
spread_std = spread.std()
zscore = (spread - spread_mean) / spread_std
# Define entry and exit thresholds for the z-score
entry_threshold = 0.8
exit_threshold = 0.2
long_signal = zscore < -entry_threshold
short_signal = zscore > entry_threshold
exit_signal = abs(zscore) < exit_threshold
positions = pd.DataFrame(index=data.index)
positions[stock1] = 0
positions[stock2] = 0
# Enter long position in stock1 and short position in stock2
positions.loc[long_signal, stock1] = 1
positions.loc[long_signal, stock2] = -1
# Enter short position in stock1 and long position in stock2
positions.loc[short_signal, stock1] = -1
positions.loc[short_signal, stock2] = 1
# Exit positions
positions.loc[exit_signal, stock1] = 0
positions.loc[exit_signal, stock2] = 0
# Calculate portfolio returns
portfolio_returns = (positions.shift(1) * (data.pct_change())).sum(axis=1)
cumulative_returns = (1 + portfolio_returns).cumprod()
return cumulative_returns, positions
For downloading this Juypter Notebook , you can follow my GitHub repository Trading_strategies
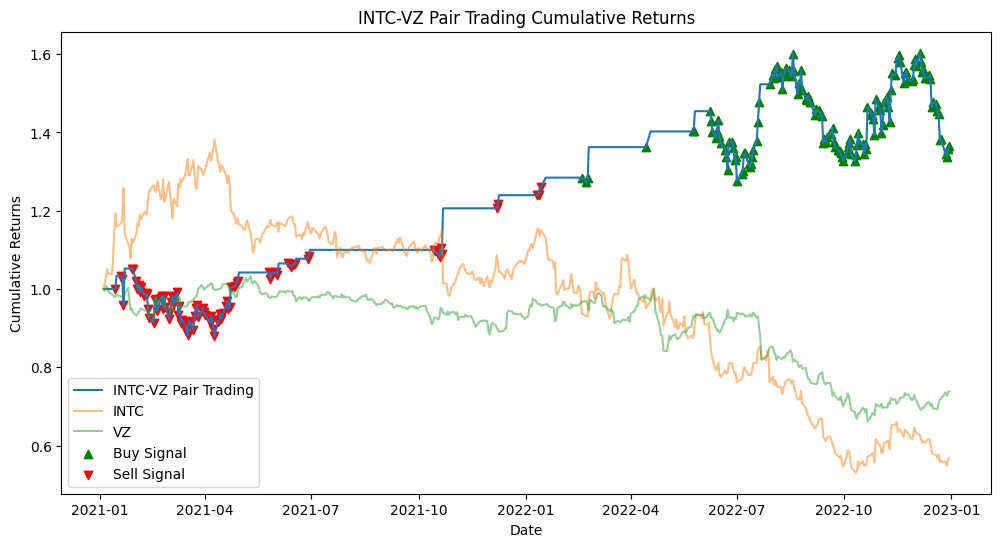
Conclusion
Pair trading is a sophisticated strategy that enables traders to benefit from the temporary deviations between co-integrated stocks. By understanding the statistical concepts of correlation and cointegration, and implementing a well-designed Python code, traders can effectively identify eligible pairs and execute profitable trades.
Pair trading is not without risks, and traders should thoroughly backtest and validate any strategy before deploying it in real markets. However, with a sound understanding of the concepts and diligent research, pair trading can offer an exciting and potentially rewarding addition to a trader’s toolbox.
Frequently Asked Questions (FAQs)
Q1: What is Pair Trading Strategy? A1: Pair trading is a statistical arbitrage strategy that involves trading two co-integrated stocks based on their historical price relationship. Traders identify pairs of stocks that historically move together and take advantage of temporary divergences from their usual relationship.
Q2: How does Pair Trading Work? A2: Pair trading involves monitoring the spread between two co-integrated stocks. When the spread diverges significantly from its historical mean, a trading signal is triggered. Traders take long or short positions depending on the direction of the divergence, aiming to profit when the spread reverts to its mean.
Q3: What is Cointegration Analysis? A3: Cointegration analysis is a statistical method used to determine whether two-time series move together in the long run, despite temporary divergences. It assesses the presence of a unit root in the spread between two stocks. A p-value less than 0.05 indicates co-integration, suggesting that deviations tend to revert to the historical mean.
Q4: How are Eligible Pairs Identified for Pair Trading? A4: Eligible pairs for pair trading are identified based on the high correlation between the stocks. The correlation measures the degree to which the stock prices move together. Additionally, cointegration analysis is performed using the Augmented Dickey-Fuller (ADF) test to confirm that the pair exhibits long-term co-movement.
Q5: How is the Pair Trading Strategy Executed? A5: The pair trading strategy is executed by calculating the z-score, which measures the number of standard deviations the spread is away from its historical mean. When the z-score exceeds a predefined entry threshold, a long or short position is taken. Subsequent positions are taken only if the previous position is closed. The strategy also includes an exit signal to close positions when the z-score approaches zero or returns change turn negative.
Q6: What is the Importance of Backtesting the Strategy? A6: Backtesting is crucial to assess the performance of the pair trading strategy using historical data. It helps traders understand how the strategy would have performed in the past, providing insights into potential risks and rewards. Backtesting allows traders to refine and optimize the strategy before deploying it in real markets.
Q7: Are There Risks Involved in Pair Trading? A7: Yes, like any trading strategy, pair trading carries risks. Market conditions, unexpected events, and changes in the relationship between stocks can impact performance. Traders should carefully manage risk, use appropriate position sizing, and conduct thorough research before implementing the strategy.
Q8: Can Pair Trading be Automated? A8: Yes, pair trading can be automated using programming languages like Python. Automated trading systems can continuously monitor eligible pairs, execute trades based on predefined rules, and manage positions based on exit signals. Automation allows for faster execution and reduces the likelihood of human errors.
Q9: Is Pair Trading Suitable for Beginners? A9: Pair trading involves statistical concepts and a thorough understanding of financial markets. While it can be suitable for beginners with proper research and guidance, beginners are advised to start with a solid foundation in trading concepts and gain experience in simpler strategies before venturing into pair trading.
Q10: What Resources are Available for Learning Pair Trading? A10: There are numerous online resources, books, and courses available for learning about pair trading. Traders can access tutorials, webinars, and research papers to understand the strategy, cointegration analysis, and Python implementation. It is essential to choose reliable sources for learning and keep up-to-date with market trends.