Trend analysis is a powerful approach in trading that allows traders to identify and analyze patterns in historical price data to make predictions about future market movements. By understanding the direction and strength of a trend, traders can make well-informed decisions on when to buy or sell assets.
In this comprehensive guide, we will delve into Python code that demonstrates trend analysis using various technical indicators and a shooting star strategy.
- Step-by-Step Guide to Implementing Trend Analysis with Python
- Importing Essential Libraries and Fetching Historical Price Data
- The Shooting Star Strategy
- Interpreting Signals: A Closer Look
- Enhancing the Strategy with Trend Analysis
- Creating a Trend Signal for Confirmation
- Identifying Uptrends and Downtrends
- Evaluating Strategy Performance through Backtesting
- Calculating Returns and Comparing against Benchmark Strategy
- Frequently Asked Questions (FAQs)
- Conclusion
Trend Analysis is a technique that involves studying historical price data to identify and interpret trends in the market. Traders utilize various technical indicators, such as moving averages, trendlines, MACD, RSI, and ATR, to identify trends and their characteristics. By understanding trends, traders can make informed decisions based on the market’s prevailing direction.
Step-by-Step Guide to Implementing Trend Analysis with Python
Before diving into Python code, it’s essential to gain a thorough understanding of the underlying concepts and techniques. The provided Python code leverages popular libraries like Pandas, yfinance, numpy, pandas_ta, and matplotlib for data manipulation, technical analysis, and visualization.
Importing Essential Libraries and Fetching Historical Price Data
To begin the trend analysis process, the code imports the necessary libraries and fetches historical price data for a specific stock (in this case, AAPL) using the yfinance library. The retrieved data is stored in a Pandas DataFrame, enabling further analysis and manipulation.
import pandas as pd
import yfinance as yf
import numpy as np
import pandas_ta as ta
import matplotlib.pyplot as plt
df = yf.download(tickers=['AAPL'],start='2020-01-01',end='2023-01-01')
print("The number of data points are", len(df))
#Check if any zero volumes are to be removed
columns = df.columns
for col in columns:
indexZeros = df[ df[col] == 0 ].index
df.drop(indexZeros , inplace=True)
The Shooting Star Strategy
The shooting star strategy is a widely used trend-based strategy that focuses on identifying potential trend reversals. The Python code implements this strategy by calculating specific conditions based on the high, low, open, and close prices, as well as the relative strength index (RSI).
The Python code incorporates the shooting star strategy by analyzing price differentials, RSI values, and trend strength. By setting certain thresholds, the code generates signals indicating potential buying or selling opportunities.
# Shooting star Strategy
alpha= 1.5
beta= 1
gamma= 1
rsi_upper_limit_buy = 90
rsi_lower_limit_buy = 50
rsi_upper_limit_sell = 40
rsi_lower_limit_sell = 10
def shooting_star_strategy(dataframe):
dataframe.dropna()
length = len(dataframe)
high = list(dataframe['High'])
low = list(dataframe['Low'])
close = list(dataframe['Close'])
open = list(dataframe['Open'])
signal = [0] * length
highdiff = [0] * length
lowdiff = [0] * length
bodydiff = [0] * length
ratio1 = [0] * length
ratio2 = [0] * length
for row in range(0, length):
highdiff[row] = high[row]-max(open[row],close[row])
bodydiff[row] = abs(open[row]-close[row])
if bodydiff[row]<0.01:
bodydiff[row]=0.01
lowdiff[row] = min(open[row],close[row])-low[row]
ratio1[row] = highdiff[row]/bodydiff[row]
ratio2[row] = lowdiff[row]/bodydiff[row]
if (ratio1[row]>alpha and lowdiff[row]<beta*highdiff[row] and bodydiff[row]>0.05 and df.RSI[row]>rsi_lower_limit_buy and df.RSI[row]<rsi_upper_limit_buy ):
signal[row] = 1
elif (ratio2[row]>alpha and highdiff[row]<beta*lowdiff[row] and bodydiff[row]>0.05 and df.RSI[row]<rsi_upper_limit_sell and df.RSI[row]>rsi_lower_limit_sell):
signal[row]=2
return signal
df['signal'] = shooting_star_strategy(df)
print("Total number of Signals Generated using Shooting Star Strategy ", (df['signal']!=0).sum())
print("Ratio of Data points to signal is ",(df['signal']!=0).sum()/len(df))
Interpreting Signals: A Closer Look
The signals generated by the shooting star strategy play a crucial role in decision-making. A signal of “1” indicates a potential buying opportunity, while a signal of “2” suggests a potential selling opportunity. These signals are derived based on specific conditions, such as price differentials, RSI thresholds, and trend strength.
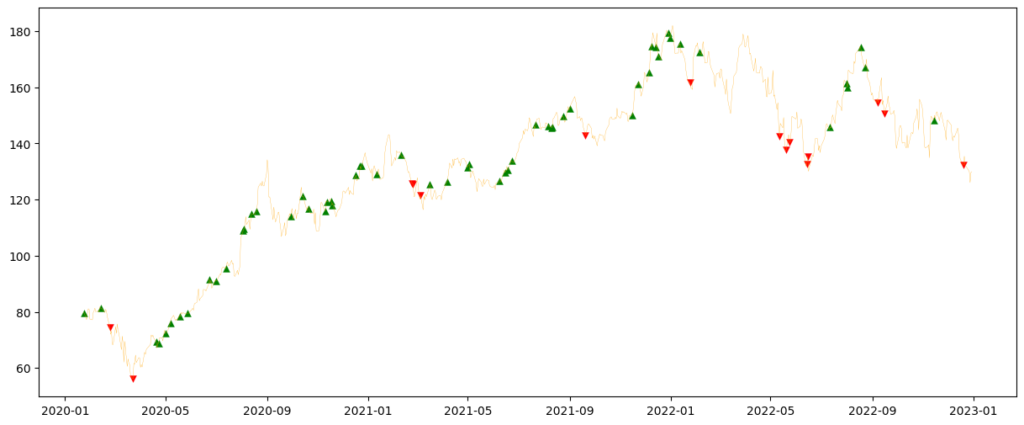
Enhancing the Strategy with Trend Analysis
To further enhance the shooting star strategy, the Python code incorporates trend analysis techniques. By utilizing the average true range (ATR) indicator and analyzing price movements, the code generates a trend signal that confirms the prevailing trend direction.
Creating a Trend Signal for Confirmation
The code calculates a trend signal by assessing the relationship between price movements and the ATR indicator. This signal provides confirmation of the prevailing trend, strengthening the trading strategy’s reliability.
#Creating Trends Signal to be used along side Stoooting Star Signal
def trend(look_ahead_period, dataframe):
length = len(dataframe)
high = list(dataframe['High'])
low = list(dataframe['Low'])
close = list(dataframe['Close'])
open = list(dataframe['Open'])
atr = list(dataframe['ATR'])
category = [0] * length
for line in range (0,length-look_ahead_period-1):
OpenLow = 0
OpenHigh = 0
highdiff = high[line]-max(open[line],close[line])
bodydiff = abs(open[line]-close[line])
y_delta = atr[line]*1. # highdiff*1.3 #for SL 400*1e-3
if y_delta<1.1:
y_delta=1.1
SLTPRatio = 2. #y_delta*Ratio gives TP
for i in range(1,look_ahead_period+1):
value1 = close[line]-low[line+i]
value2 = close[line]-high[line+i]
OpenLow = max(value1, OpenLow)
OpenHigh = min(value2,OpenHigh)
if ( (OpenLow >= (SLTPRatio*y_delta) ) and (-OpenHigh < y_delta) ):
category[line] = 1 #-1 downtrend
break
elif ((OpenLow < y_delta) ) and (-OpenHigh >= (SLTPRatio*y_delta)):
category[line] = 2 # uptrend
break
else:
category[line] = 0 # no clear trend
return category
# trend(barsfront to take into account, dataframe)
df['Trend'] = trend(100, df)
Identifying Uptrends and Downtrends
By analyzing the trend signal, traders can identify different types of trends, including uptrends and downtrends. These insights assist in aligning trading decisions with the overall market direction.
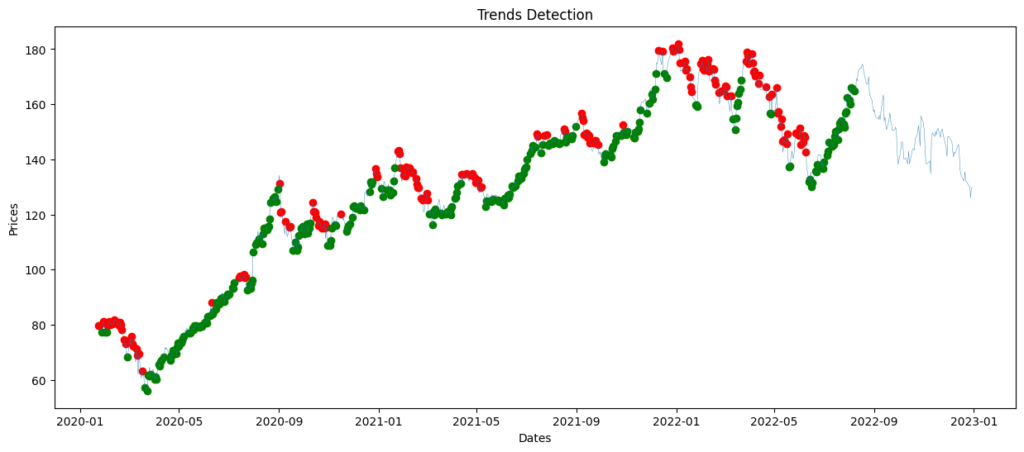
Evaluating Strategy Performance through Backtesting
The Python code evaluates the performance of the implemented trend analysis strategy through backtesting. Backtesting involves testing the strategy on historical data to assess its effectiveness and potential profitability.
Calculating Returns and Comparing against Benchmark Strategy
The code calculates the returns generated by the trend analysis strategy. By comparing the strategy’s returns against a buy-and-hold strategy, traders can gain insights into its profitability and risk-reward profile.
df['Positions'] = 0
df['Positions'].loc[df['Trend'] ==1] = -1
df['Positions'].loc[df['Trend'] ==2] = 1
df['Strat_ret'] = df['Positions'].diff().shift(1) * df['Ret']
df['Positions_L'] = df['Positions'].diff().shift(1)
df.loc[df['Positions_L'] == -1, 'Positions_L'] = 0
df['Strat_ret_L'] = df['Positions_L'] * df['Ret']
df['CumRet'] = (1 + df['Strat_ret']).cumprod() - 1
df['CumRet_L'] = (1 + df['Strat_ret_L']).cumprod() - 1
df['bhRet'] = df['Ret'].cumsum()
Final_Return_L = np.prod(1 + df["Strat_ret_L"]) - 1
Final_Return = np.prod(1 + df["Strat_ret"]) - 1
Buy_Return = np.prod(1 + df["Ret"]) - 1
print("Strat Return Long Only =", Final_Return_L * 100, "%")
print("Strat Return =", Final_Return * 100, "%")
print("Buy and Hold Return =", Buy_Return * 100, "%")
fig = plt.figure(figsize=(12, 6))
ax = plt.gca()
df.plot(x="Date", y="bhRet", label=f"Buy & Hold Only {Buy_Return.round(3)*100} % Returns ", ax=ax)
df.plot(x="Date", y="CumRet_L", label=f"Taking Only Long from Strategy {Final_Return_L.round(3)*100} % Returns ", ax=ax)
df.plot(x="Date", y="CumRet", label=f"Taking Both Long Short from Strategy {Final_Return.round(3)*100} % Returns ", ax=ax)
plt.xlabel("date")
plt.ylabel("Cumulative Returns")
plt.grid()
plt.show()
The code provides a comparison between the trend analysis strategy’s returns and the returns of a buy-and-hold strategy. This analysis helps traders gauge the strategy’s effectiveness and determine whether it outperforms a simple buy-and-hold approach.
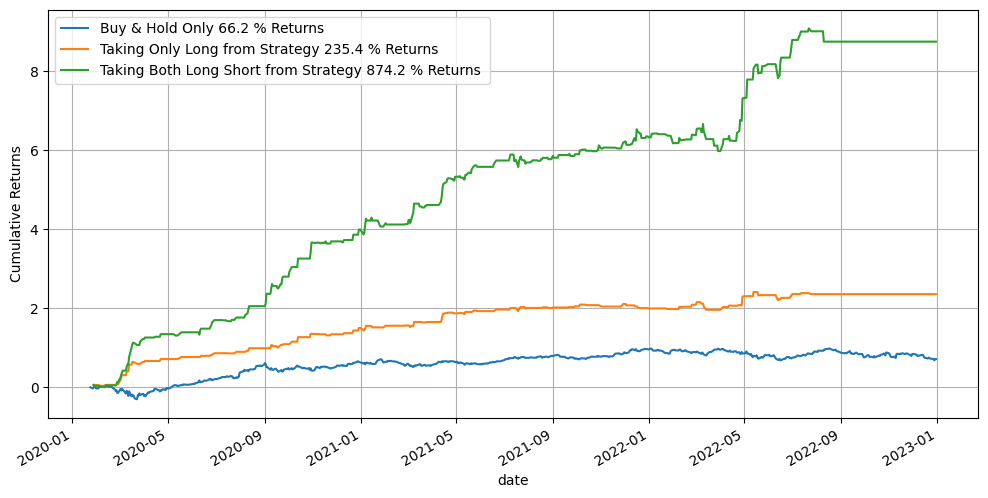
For more such strategies visit my gihub repo
Frequently Asked Questions (FAQs)
Q1: Why is trend analysis important in trading, and how can it help traders improve their decision-making process?
- Answer: Trend analysis is crucial in trading as it enables traders to identify and follow market trends, enhancing their decision-making process. By understanding trends, traders can determine the optimal timing for buying or selling assets, improving their trading outcomes.
Q2: What are some commonly used technical indicators for trend analysis, and how do they work?
- Answer: Popular technical indicators for trend analysis include moving averages, which smooth out price data and highlight trend direction, and the relative strength index (RSI), which measures the speed and change of price movements. These indicators provide insights into trend strength and potential reversals.
Q3: How can traders interpret the signals generated by the shooting star strategy?
- Answer: The shooting star strategy generates signals indicating potential buying or selling opportunities. A signal of “1” suggests a potential buying opportunity, while a signal of “2” indicates a potential selling opportunity. Traders should consider these signals alongside other factors, such as volume and overall market conditions, to make informed decisions.
Q4: How does backtesting assist in evaluating the performance of a trading strategy?
- Answer: Backtesting involves applying a trading strategy to historical data to simulate how it would have performed in the past. By comparing the strategy’s returns, risk metrics, and other performance indicators against benchmarks like a buy-and-hold strategy, traders can assess its effectiveness and refine their approach.
Conclusion
Trend analysis is a valuable tool in trading that empowers traders to capitalize on market trends. By leveraging Python and the provided code, beginners can gain a solid understanding of the trend analysis process and its practical implementation in a trading strategy. The detailed explanations, along with FAQs, simplify complex jargon and make trend analysis accessible to traders at all skill levels.
Through continuous learning, exploration, and experimentation, traders can refine their trend analysis strategies and improve their trading skills. Always remember to conduct thorough research, test different strategies, and practice proper risk management techniques when applying any trading strategy.
Note: This comprehensive guide offers a detailed overview of trend analysis and its implementation in trading. As you progress, continue exploring more advanced concepts to further enhance your trading abilities.