Algorithmic trading has revolutionized the financial markets, empowering traders to execute strategies with precision and speed. Among the numerous indicators used in algorithmic trading, the Relative Strength Index (RSI) stands out as a powerful tool for making informed trading decisions. In this comprehensive guide, we will backtest RSI strategy using Python. We’ll not only dive deep into the code but also provide insights into different indicators and combinations that can enhance your trading arsenal.
Understanding the RSI Indicator
The Relative Strength Index (RSI) is a momentum oscillator that measures the speed and change of price movements. It ranges from 0 to 100 and is typically used to identify overbought and oversold conditions in an asset. Here’s a step-by-step breakdown of how the RSI is calculated
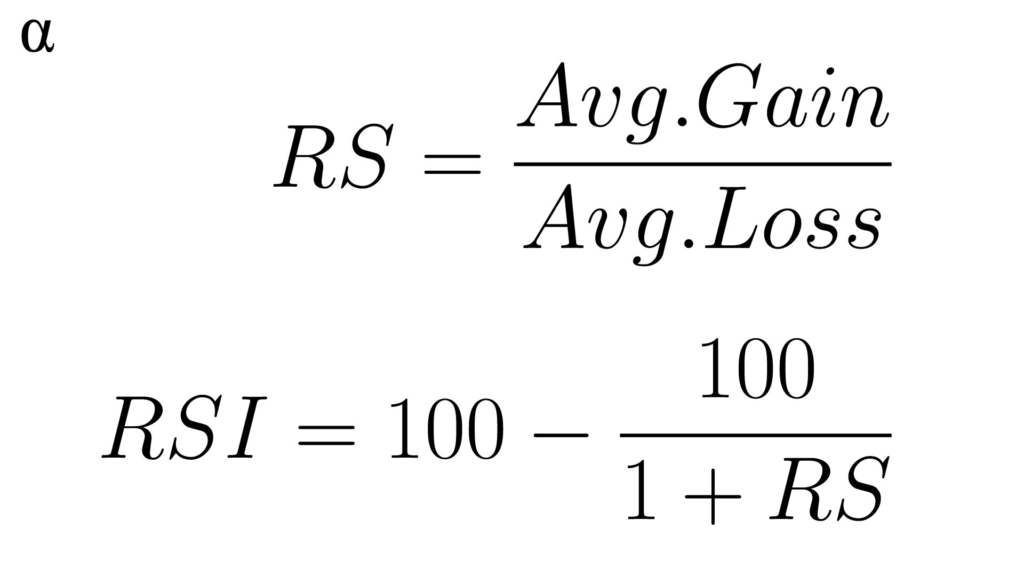
- Calculate the daily price change by subtracting the previous day’s closing price from the current day’s closing price.
- Separate positive price changes (gains) and negative price changes (losses). Set negative changes to zero and positive changes to their absolute values.
- Calculate the average gain and average loss over a specified period (commonly 14 days).
- Calculate the Relative Strength (RS) by dividing the average gain by the average loss.
- Calculate the RSI using the formula: RSI = 100 – (100 / (1 + RS))
Also Read Trading Strategies using Machine Learning: A Guide to K-Nearest Neighbors (KNN) Classifier
Fetching and Preprocessing Data to Backtest RSI Strategy
Before implementing the RSI-based strategy, we need historical price data for an asset. We’ll use the Yahoo Finance API to fetch daily price data for Mahindra and Mahindra (M&M) as an example.
import yfinance as yf
from datetime import datetime
# Load the data into a dataframe
ticker = ['M&M.NS']
df = yf.download(tickers=ticker, interval="1d", period="max")
# Filter the data by date
df_window = df[df.index > datetime(2020, 1, 1)]
df_window = df[df.index < datetime(2021, 9, 1)]
Calculating the RSI
With the data loaded, we can proceed to calculate the RSI. We’ll use a rolling window of 14 days for our RSI calculations.
change = df["Close"].diff()
change.dropna(inplace=True)
change_up = change.copy()
change_down = change.copy()
change_up[change_up < 0] = 0
change_down[change_down > 0] = 0
window = 14
avg_up = change_up.rolling(window=window).mean()
avg_down = change_down.rolling(window=window).mean().abs()
rsi = 100 * avg_up / (avg_up + avg_down)
rsi_dropna = rsi.dropna()
Visualizing the RSI
To gain insights from the RSI, we’ll visualize it alongside the asset’s closing price. This visual representation helps identify potential buy and sell signals.
import matplotlib.pyplot as plt
# Set the theme of our chart
plt.style.use('fivethirtyeight')
# Create two charts on the same figure
ax1 = plt.subplot2grid((10, 1), (0, 0), rowspan=4, colspan=1)
ax2 = plt.subplot2grid((10, 1), (5, 0), rowspan=4, colspan=1)
# First chart:
# Plot the closing price on the first chart
ax1.plot(df['Close'], linewidth=2)
ax1.set_title('Mahindra and Mahindra Close Price')
# Second chart
# Plot the RSI
ax2.set_title('Relative Strength Index')
ax2.plot(rsi, color='orange', linewidth=1)
# Add horizontal lines for buy and sell ranges
ax2.axhline(30, linestyle='--', linewidth=1.5, color='green') # Oversold
ax2.axhline(70, linestyle='--', linewidth=1.5, color='red') # Overbought
# Display the charts
plt.show()
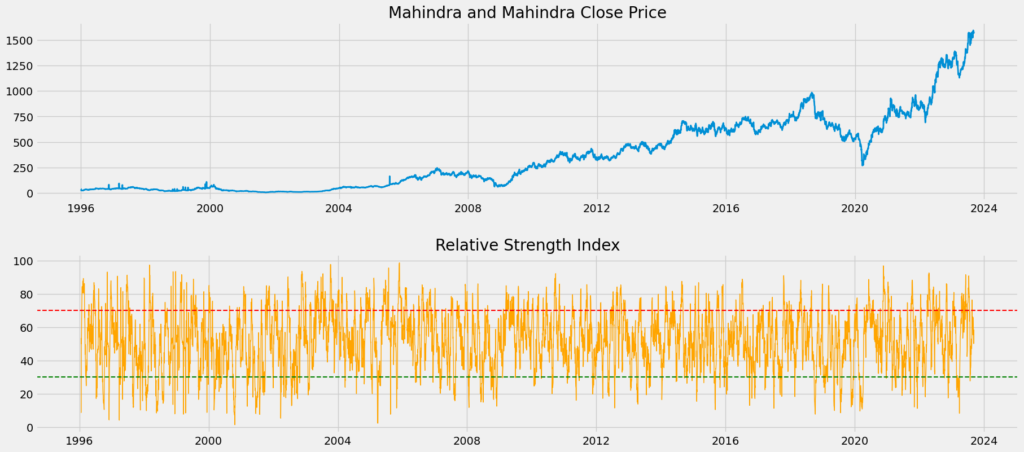
Implementing an RSI Strategy
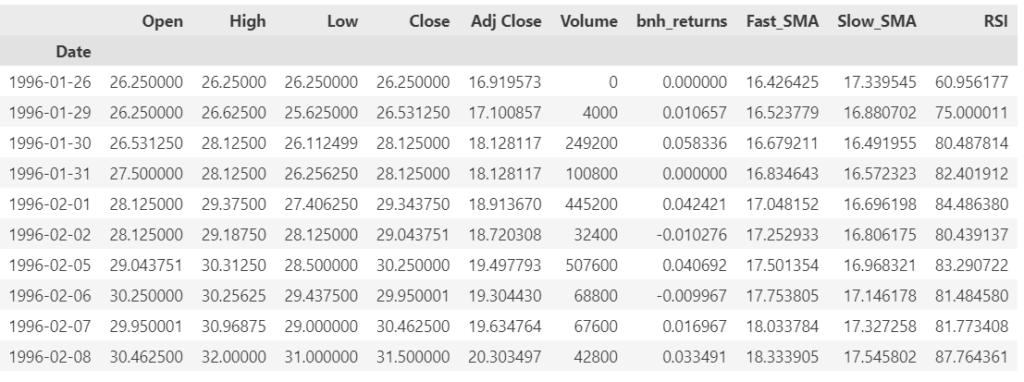
The RSI can be a powerful tool when integrated into a trading strategy. Here, we provide an example of an RSI-based strategy:
- Long Signal: Buy when RSI is below 60, and the Fast Simple Moving Average (SMA) crosses above the Slow SMA.
- Short Signal: Sell when RSI is above 70, and the Fast SMA crosses below the Slow SMA.
fast_window = 12 slow_window = 20
fast_window = 12 slow_window = 20 # Long Signal df['signal'] = np.where((df['RSI'] < 60) & (df['Fast_SMA'] > df['Slow_SMA']) & (df['Fast_SMA'].shift(1) <= df['Slow_SMA']), 1, 0) # Short Signal df['signal'] = np.where((df['RSI'] > 70) & (df['Fast_SMA'] < df['Slow_SMA']) & (df['Slow_SMA'].shift(1) <= df['Slow_SMA']), -1, df['signal'])# Signal Matrix # Long Signal df['signal'] = np.where((df['RSI'] < 60) & (df['Fast_SMA'] > df['Slow_SMA']) & (df['Fast_SMA'].shift(1) <= df['Slow_SMA']), 1, 0) # Short Signal df['signal'] = np.where((df['RSI'] > 70) & (df['Fast_SMA'] < df['Slow_SMA']) & (df['Slow_SMA'].shift(1) <= df['Slow_SMA']), -1, df['signal'])
Evaluating Strategy Performance
After implementing the RSI-based strategy, it’s essential to assess its performance. We can compare it with a simple buy-and-hold strategy to gauge its effectiveness.
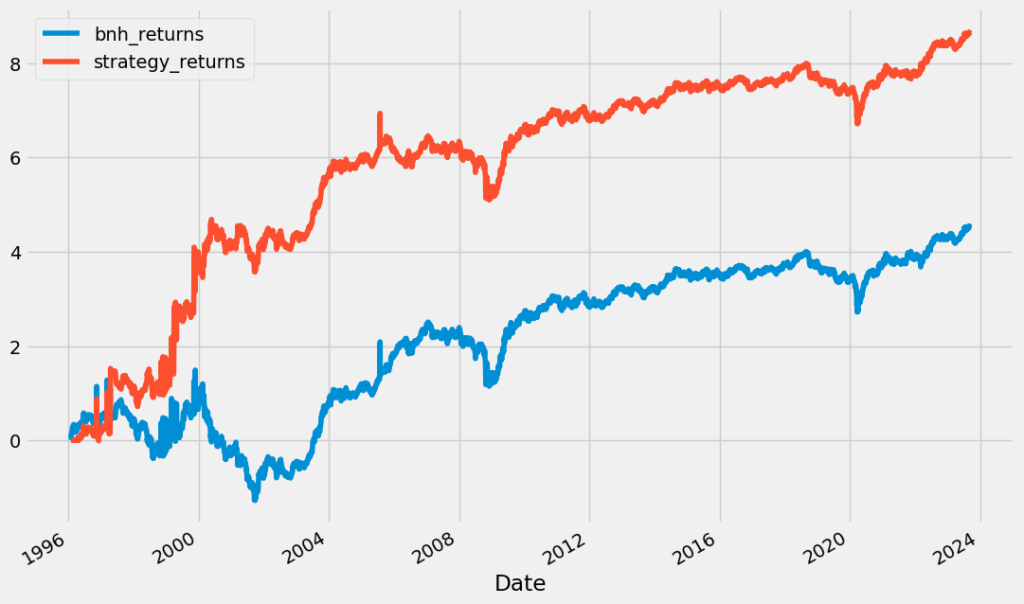
# comparing buy & hold strategy / RSI strategy returns
print("Buy and hold returns:", df['bnh_returns'].cumsum()[-1])
print("Strategy returns:", df['strategy_returns'].cumsum()[-1])
# plotting strategy historical performance over time
df[['bnh_returns', 'strategy_returns']].plot(grid=True, figsize=(12, 8))
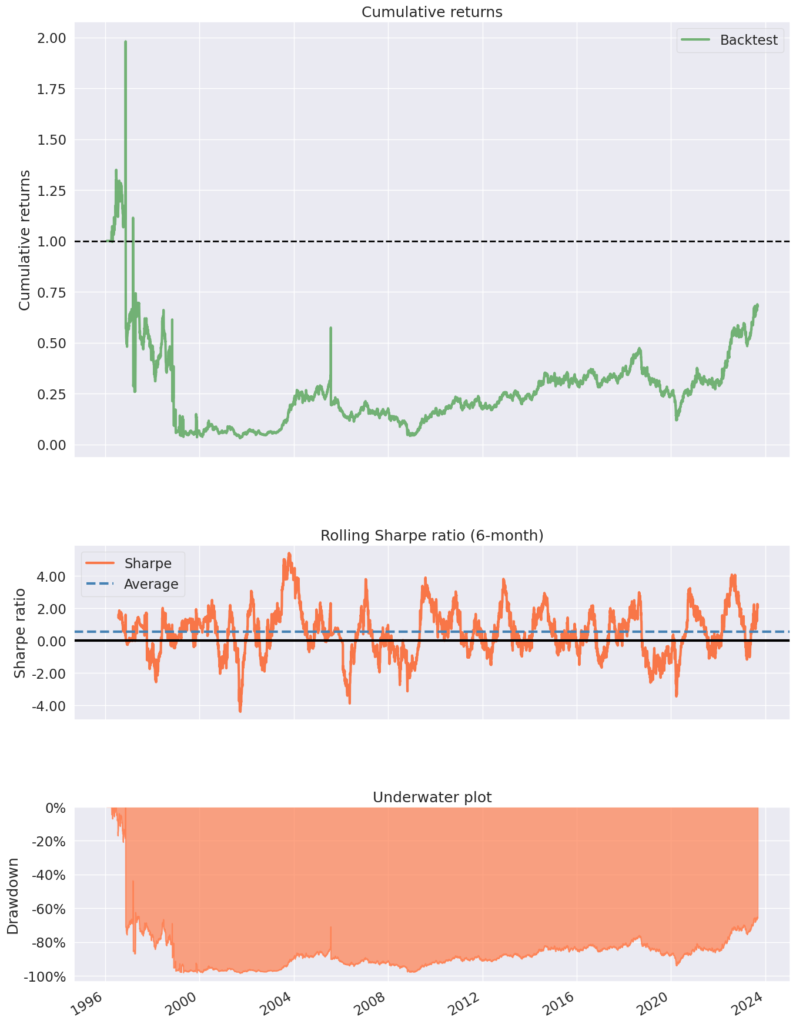
Additional Indicator Combinations
To enhance your trading strategies, consider combining the RSI with other indicators:
- Moving Averages (e.g., Simple Moving Average, Exponential Moving Average)
- Bollinger Bands
- MACD (Moving Average Convergence Divergence)
- Stochastic Oscillator
These combinations can provide a more comprehensive view of market conditions and potential entry or exit points.
To Download Complete notebook, visit our GitHub repository -> QuantifiedTrader
Conclusion
In this comprehensive guide, we’ve explored the Relative Strength Index (RSI) and its application in algorithmic trading using Python. The RSI is a versatile tool that can help traders identify potential buy and sell signals. While we’ve demonstrated one RSI-based strategy, there are numerous indicators and combinations that traders can explore to enhance their trading strategies further.
As a trader or investor, mastering technical indicators like the RSI can be a valuable asset in navigating the complexities of financial markets. Whether you choose to use RSI alone or in combination with other indicators, understanding its principles is a crucial step toward becoming a successful algorithmic trader
FAQs
What is the RSI indicator?
The Relative Strength Index (RSI) is a momentum oscillator used to measure the speed and magnitude of price changes. It helps traders identify overbought and oversold conditions in an asset.
Can I use the RSI alone in my trading strategy?
While the RSI is a powerful indicator, combining it with other indicators such as moving averages, Bollinger Bands, MACD, and stochastic oscillators can provide a more comprehensive trading strategy.
How can I assess the performance of my RSI-based strategy?
You can evaluate your strategy’s performance by comparing it to a buy-and-hold strategy. Calculate and compare returns over time to determine its effectiveness.
Are there specific RSI values for buy and sell signals?
Commonly, an RSI below 30 indicates oversold conditions (a potential buy signal), while an RSI above 70 suggests overbought conditions (a potential sell signal). However, these thresholds can be adjusted based on your strategy and assets.
What programming language is suitable for algorithmic trading?
Python is a popular choice for algorithmic trading due to its versatility, extensive libraries, and readability.
This guide equips you with the knowledge and tools to implement RSI-based strategies in Python. Remember that successful trading requires continuous practice, risk management, and adaptation to changing market conditions.